SDK及代碼示例:快遞單號(hào)智能識(shí)別/快遞公司+快遞單號(hào)查詢接口API
1、PHP SDK
1.1、接口一:快遞單號(hào)智能識(shí)別
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//接口參數(shù) $api_url='http://cha.ebaitian.cn/api/json'; $api_appid='1000xxxx'; $api_appkey='56cf61af4b7897e704f67deb88ae8f24'; //函數(shù),以POST方式提交數(shù)據(jù),PHP需要開啟CURL函數(shù);數(shù)據(jù)傳輸安全,建議使用 function getExpressInfo($order){ global $api_url,$api_appid,$api_appkey; $posturl=$api_url; $data='appid='.$api_appid.'&module=getExpressInfo&order='.$order; $sign=hash("sha256",$data.'&appkey='.$api_appkey); $postdata=array("appid"=>$api_appid,"appkey"=>$api_appkey,"module"=>"getExpressInfo","order"=>$order,'sign'=>$sign); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $posturl); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $output = curl_exec($curl); curl_close($curl); $obj=json_decode($output); $result=$obj->result; if($result==1){ $value=$obj->expressInfo->name; $value.=','.$obj->expressInfo->order; $value.=','.$obj->expressInfo->status; }else{ $value=$obj->flag; } return $value; } //調(diào)用函數(shù) $order='247198050163'; echo getExpressInfo($order); exit;
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//接口參數(shù) $api_url='http://cha.ebaitian.cn/api/json'; $api_appid='1000xxxx'; $api_appkey='56cf61af4b7897e704f67deb88ae8f24'; //函數(shù),以GET方式提交數(shù)據(jù) function getExpressInfo($order){ global $api_url,$api_appid,$api_appkey; $data='appid='.$api_appid.'&module=getExpressInfo&order='.$order; $sign=hash("sha256",$data.'&appkey='.$api_appkey); $info_get=file_get_contents($api_url.'?type=get&'.$data.'&sign='.$sign); $info_json=json_decode($info_get, true); $result=$info_json['result']; if($result==1){ $value=$info_json['expressInfo']['name']; $value.=','.$info_json['expressInfo']['order']; $value.=','.$info_json['expressInfo']['status']; }else{ $value=$info_json['flag']; } return $value; } //調(diào)用函數(shù) $order='247198050163'; echo getExpressInfo($order); exit;
1.2、接口二:快遞公司+快遞單號(hào)
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//接口參數(shù) $api_url='http://cha.ebaitian.cn/api/json'; $api_appid='1000xxxx'; $api_appkey='56cf61af4b7897e704f67deb88ae8f24'; //函數(shù),以POST方式提交數(shù)據(jù),PHP需要開啟CURL函數(shù);數(shù)據(jù)傳輸安全,建議使用 function getExpressInfo($company,$order){ global $api_url,$api_appid,$api_appkey; $posturl=$api_url; $data='appid='.$api_appid.'&module=getExpressInfoNoSmart&company=company&order='.$order; $sign=hash("sha256",$data.'&appkey='.$api_appkey); $postdata=array("appid"=>$api_appid,"appkey"=>$api_appkey,"module"=>"getExpressInfoNoSmart","company"=>$company,"order"=>$order,'sign'=>$sign); $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $posturl); curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1); curl_setopt($curl, CURLOPT_POST, 1); curl_setopt($curl, CURLOPT_POSTFIELDS, $postdata); $output = curl_exec($curl); curl_close($curl); $obj=json_decode($output); $result=$obj->result; if($result==1){ $value=$obj->expressInfo->name; $value.=','.$obj->expressInfo->order; $value.=','.$obj->expressInfo->status; }else{ $value=$obj->flag; } return $value; } //調(diào)用函數(shù) $order='247198050163'; echo getExpressInfo($order); exit;
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//接口參數(shù) $api_url='http://cha.ebaitian.cn/api/json'; $api_appid='1000xxxx'; $api_appkey='56cf61af4b7897e704f67deb88ae8f24'; //函數(shù),以GET方式提交數(shù)據(jù) function getExpressInfo($company,$order){ global $api_url,$api_appid,$api_appkey; $data='appid='.$api_appid.'&module=getExpressInfoNoSmart&company=$company&order='.$order; $sign=hash("sha256",$data.'&appkey='.$api_appkey); $info_get=file_get_contents($api_url.'?type=get&'.$data.'&sign='.$sign); $info_json=json_decode($info_get, true); $result=$info_json['result']; if($result==1){ $value=$info_json['expressInfo']['name']; $value.=','.$info_json['expressInfo']['order']; $value.=','.$info_json['expressInfo']['status']; }else{ $value=$info_json['flag']; } return $value; } //調(diào)用函數(shù) $order='247198050163'; echo getExpressInfo($company,$order); exit;
2、Java SDK
2.1、接口一:快遞單號(hào)智能識(shí)別
//以下示例是以 GET 方式請(qǐng)求數(shù)據(jù) public class QueryHelper { public static String apiurl="http://cha.ebaitian.cn/api/json"; public static String appid="1000xxxx"; public static String appkey="56cf61af4b7897e704f67deb88ae8f24"; public static String module="getExpressInfo"; public static String getSHA256Str(String str){ MessageDigest messageDigest; String encdeStr = ""; try { messageDigest = MessageDigest.getInstance("SHA-256"); byte[] hash = messageDigest.digest(str.getBytes("UTF-8")); encdeStr = Hex.encodeHexString(hash); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return encdeStr; } public static String get(String urlString) { try { URL url = new URL(urlString); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setConnectTimeout(5 * 1000); conn.setReadTimeout(5 * 1000); conn.setDoInput(true); conn.setDoOutput(true); conn.setUseCaches(false); conn.setInstanceFollowRedirects(false); conn.setRequestMethod("GET"); int responseCode = conn.getResponseCode(); if (responseCode == 200) { StringBuilder builder = new StringBuilder(); BufferedReader br = new BufferedReader( new InputStreamReader(conn.getInputStream(),"utf-8")); for (String s = br.readLine(); s != null; s = br.readLine()) { builder.append(s); } br.close(); return builder.toString(); } } catch (IOException e) { e.printStackTrace(); } return null; } public static String queryExpress(String order){ String sign=getSHA256Str("appid="+appid+"&module="+module+"&order="+order+"&appkey="+appkey); String url=apiurl+"?type=get&appid="+appid+"&module="+module+"&order="+order+"&sign="+sign; return get(url); } } //使用示例 QueryHelper.queryExpress("247198050163");
2.2、接口二:快遞公司+快遞單號(hào)
//以下示例是以 GET 方式請(qǐng)求數(shù)據(jù) public class QueryHelper { public static String apiurl="http://cha.ebaitian.cn/api/json"; public static String appid="1000xxxx"; public static String appkey="56cf61af4b7897e704f67deb88ae8f24"; public static String module="getExpressInfoNoSmart"; public static String getSHA256Str(String str){ MessageDigest messageDigest; String encdeStr = ""; try { messageDigest = MessageDigest.getInstance("SHA-256"); byte[] hash = messageDigest.digest(str.getBytes("UTF-8")); encdeStr = Hex.encodeHexString(hash); } catch (NoSuchAlgorithmException e) { e.printStackTrace(); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } return encdeStr; } public static String get(String urlString) { try { URL url = new URL(urlString); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setConnectTimeout(5 * 1000); conn.setReadTimeout(5 * 1000); conn.setDoInput(true); conn.setDoOutput(true); conn.setUseCaches(false); conn.setInstanceFollowRedirects(false); conn.setRequestMethod("GET"); int responseCode = conn.getResponseCode(); if (responseCode == 200) { StringBuilder builder = new StringBuilder(); BufferedReader br = new BufferedReader( new InputStreamReader(conn.getInputStream(),"utf-8")); for (String s = br.readLine(); s != null; s = br.readLine()) { builder.append(s); } br.close(); return builder.toString(); } } catch (IOException e) { e.printStackTrace(); } return null; } public static String queryExpress(String company, String order){ String sign=getSHA256Str("appid="+appid+"&module="+module+"&company="+company+"&order="+order+"&appkey="+appkey); String url=apiurl+"?type=get&appid="+appid+"&module="+module+"&company="+company+"&order="+order+"&sign="+sign; return get(url); } } //使用示例 QueryHelper.queryExpress("shunfeng", "247198050163");
3、Python SDK
3.1、接口一:快遞單號(hào)智能識(shí)別
#!/usr/bin/python # -*- coding: utf-8 -*- import httplib2 import hashlib from urllib.parse import urlencode #python3 #from urllib import urlencode #python2 apiurl='http://cha.ebaitian.cn/api/json' appid='1000xxxx' appkey='56cf61af4b7897e704f67deb88ae8f24' module='getExpressInfo' order='247198050163' data='appid='+appid+'&module='+module+'&order='+order sign_data=data+'&appkey='+appkey # from Crypto.Cipher import AES # from Crypto.Hash import SHA256 # 256 hash_256 = hashlib.sha256() hash_256.update(sign_data.encode('utf-8')) sign = hash_256.hexdigest() postdata = urlencode({'appid':appid,'module':module,'order':order,'sign':sign}) url = apiurl+'?'+postdata http = httplib2.Http() response, content = http.request(url,'GET') print(content.decode("utf-8"))
3.2、接口二:快遞公司+快遞單號(hào)
#!/usr/bin/python # -*- coding: utf-8 -*- import httplib2 import hashlib from urllib.parse import urlencode #python3 #from urllib import urlencode #python2 apiurl='http://cha.ebaitian.cn/api/json' appid='1000xxxx' appkey='56cf61af4b7897e704f67deb88ae8f24' module='getExpressInfoNoSmart' company='shunfeng' order='247198050163' data='appid='+appid+'&module='+module+'&company='+company+'&order='+order sign_data=data+'&appkey='+appkey # from Crypto.Cipher import AES # from Crypto.Hash import SHA256 # 256 hash_256 = hashlib.sha256() hash_256.update(sign_data.encode('utf-8')) sign = hash_256.hexdigest() postdata = urlencode({'appid':appid,'module':module,'company':company,'order':order,'sign':sign}) url = apiurl+'?'+postdata http = httplib2.Http() response, content = http.request(url,'GET') print(content.decode("utf-8"))
4、Node.js SDK
4.1、接口一:快遞單號(hào)智能識(shí)別
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//以 POST 方式提交 var http = require('http'); var querystring = require('querystring'); //參數(shù)設(shè)置 var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfo'; //目標(biāo)查詢快遞單號(hào) var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&order='+order+'&appkey='+appkey); //這是需要提交的數(shù)據(jù) var post_data = { appid: appid, module: module, order: order, sign: sign }; var content = querystring.stringify(post_data); var options = { hostname: 'cha.ebaitian.cn', port: 80, path: '/api/json', method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8' } }; var req = http.request(options, function (res) { console.log('STATUS: ' + res.statusCode); console.log('HEADERS: ' + JSON.stringify(res.headers)); res.setEncoding('utf8'); res.on('data', function (chunk) { console.log('BODY: ' + chunk); //JSON.parse(chunk) }); }); req.on('error', function (e) { console.log('problem with request: ' + e.message); }); // write data to request body req.write(content); req.end();
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//以 GET 方式提交 var http = require('http'); var querystring = require('querystring'); //參數(shù)設(shè)置 var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfo'; //目標(biāo)查詢快遞單號(hào) var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&order='+order+'&appkey='+appkey); //這是需要提交的數(shù)據(jù) var data = { appid: appid, module: module, order: order, sign: sign }; var content = querystring.stringify(data); var options = { hostname: 'cha.ebaitian.cn', port: 80, path: '/api/json?' + content, method: 'GET' }; var req = http.request(options, function (res) { console.log('STATUS: ' + res.statusCode); console.log('HEADERS: ' + JSON.stringify(res.headers)); res.setEncoding('utf8'); res.on('data', function (chunk) { console.log('BODY: ' + chunk); }); }); req.on('error', function (e) { console.log('problem with request: ' + e.message); }); req.end();
4.2、接口二:快遞公司+快遞單號(hào)
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//以 POST 方式提交 var http = require('http'); var querystring = require('querystring'); //參數(shù)設(shè)置 var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfoNoSmart'; //目標(biāo)查詢快遞公司及快遞單號(hào) var company='shunfeng'; var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&company='+company+'&order='+order+'&appkey='+appkey); //這是需要提交的數(shù)據(jù) var post_data = { appid: appid, module: module, company: company, order: order, sign: sign }; var content = querystring.stringify(post_data); var options = { hostname: 'cha.ebaitian.cn', port: 80, path: '/api/json', method: 'POST', headers: { 'Content-Type': 'application/x-www-form-urlencoded; charset=UTF-8' } }; var req = http.request(options, function (res) { console.log('STATUS: ' + res.statusCode); console.log('HEADERS: ' + JSON.stringify(res.headers)); res.setEncoding('utf8'); res.on('data', function (chunk) { console.log('BODY: ' + chunk); //JSON.parse(chunk) }); }); req.on('error', function (e) { console.log('problem with request: ' + e.message); }); // write data to request body req.write(content); req.end();
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//以 GET 方式提交 var http = require('http'); var querystring = require('querystring'); //參數(shù)設(shè)置 var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfoNoSmart'; //目標(biāo)查詢快遞公司及快遞單號(hào) var company='shunfeng'; var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&company='+company+'&order='+order+'&appkey='+appkey); //這是需要提交的數(shù)據(jù) var data = { appid: appid, module: module, company: company, order: order, sign: sign }; var content = querystring.stringify(data); var options = { hostname: 'cha.ebaitian.cn', port: 80, path: '/api/json?' + content, method: 'GET' }; var req = http.request(options, function (res) { console.log('STATUS: ' + res.statusCode); console.log('HEADERS: ' + JSON.stringify(res.headers)); res.setEncoding('utf8'); res.on('data', function (chunk) { console.log('BODY: ' + chunk); }); }); req.on('error', function (e) { console.log('problem with request: ' + e.message); }); req.end();
5、C# SDK
5.1、接口一:快遞單號(hào)智能識(shí)別
using System; using System.Collections.Generic; using System.Web; using System.Net; using System.Text; public class getMobileInfo{ public static string getInfo(string appid, string appkey, string module, string order){ string url = string.Format("http://cha.ebaitian.cn/api/json?type=get&appid={0}&module={1}&order={2}&sgin={3}", appid, module, order, sgin); using (WebClient client = new WebClient()){ client.Encoding = Encoding.UTF8; return client.DownloadString(url); } } } string expressInfo = getMobileInfo.getInfo("1000xxxx", "getExpressInfo", "247198050163", "ecab4881ee80ad3d76bb1da68387428ca752eb885e52621a3129dcf4d9bc4fd4", Request.UserHostAddress); Console.WriteLine(expressInfo); Response.Write(expressInfo);
5.2、接口二:快遞公司+快遞單號(hào)
using System; using System.Collections.Generic; using System.Web; using System.Net; using System.Text; public class getMobileInfo{ public static string getInfo(string appid, string appkey, string module, string company, string order){ string url = string.Format("http://cha.ebaitian.cn/api/json?type=get&appid={0}&module={1}&company={2}&order={3}&sgin={4}", appid, module, company, order, sgin); using (WebClient client = new WebClient()){ client.Encoding = Encoding.UTF8; return client.DownloadString(url); } } } string expressInfo = getMobileInfo.getInfo("1000xxxx", "getExpressInfoNoSmart", "shunfeng", "247198050163", "ecab4881ee80ad3d76bb1da68387428ca752eb885e52621a3129dcf4d9bc4fd4", Request.UserHostAddress); Console.WriteLine(expressInfo); Response.Write(expressInfo);
6、JavaScript SDK
6.1、接口一:快遞單號(hào)智能識(shí)別
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//使用 JQuery 請(qǐng)先加載最新的 JQuery 插件 //參數(shù)設(shè)置 var apiurl = 'http://cha.ebaitian.cn/api/json'; var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfo'; //目標(biāo)查詢快遞單號(hào) var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&order='+order+'&appkey='+appkey); //提交數(shù)據(jù) $.ajax({ url:apiurl, type:'post', dataType:'json', data:{ appid:appid, module:module, order:order, sign:sign }, success:function(res){ console.log(res); } });
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//使用 JQuery 請(qǐng)先加載最新的 JQuery 插件 //參數(shù)設(shè)置 var apiurl = 'http://cha.ebaitian.cn/api/json'; var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfo'; //目標(biāo)查詢快遞單號(hào) var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&order='+order+'&appkey='+appkey); //提交數(shù)據(jù) $.ajax({ url:apiurl, type:'post', dataType:'json', data:{ appid:appid, module:module, order:order, sign:sign }, success:function(res){ console.log(res); } });
6.2、接口二:快遞公司+快遞單號(hào)
方法一:以 POST 方式請(qǐng)求數(shù)據(jù)
//使用 JQuery 請(qǐng)先加載最新的 JQuery 插件 //參數(shù)設(shè)置 var apiurl = 'http://cha.ebaitian.cn/api/json'; var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfoNoSmart'; //目標(biāo)查詢快遞公司及快遞單號(hào) var company='shunfeng'; var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&company='+company+'&order='+order+'&appkey='+appkey); //提交數(shù)據(jù) $.ajax({ url:apiurl, type:'post', dataType:'json', data:{ appid:appid, module:module, company:company, order:order, sign:sign }, success:function(res){ console.log(res); } });
方法二:以 GET 方式請(qǐng)求數(shù)據(jù)
//使用 JQuery 請(qǐng)先加載最新的 JQuery 插件 //參數(shù)設(shè)置 var apiurl = 'http://cha.ebaitian.cn/api/json'; var appid = '1000xxxx'; var appkey = '56cf61af4b7897e704f67deb88ae8f24'; var module = 'getExpressInfoNoSmart'; //目標(biāo)查詢快遞公司及快遞單號(hào) var company='shunfeng'; var order='247198050163'; //簽名,SHA256 不可直接調(diào)用;函數(shù)參考下載地址:https://github.com/alexweber/jquery.sha256 var sign = SHA256('appid='+appid+'&module='+module+'&company='+company+'&order='+order+'&appkey='+appkey); //提交數(shù)據(jù) $.ajax({ url:apiurl, type:'post', dataType:'json', data:{ appid:appid, module:module, company:company, order:order, sign:sign }, success:function(res){ console.log(res); } });
7、ASP SDK
7.1、接口一:快遞單號(hào)智能識(shí)別
'設(shè)置參數(shù) dim apiurl, appid, appkey, module, order, sign apiurl="http://cha.ebaitian.cn/api/json" appid="1000xxxx' appkey="56cf61af4b7897e704f67deb88ae8f24" module="getExpressInfo" order="247198050163" '簽名,SHA256 不可直接調(diào)用;函數(shù)參考地址:https://blog.csdn.net/yesoce/article/details/128546 sgin=SHA256("appid=&appid&"&module="&module&"&order="&order&"&appkey="&appkey) '異步提交數(shù)據(jù) function PostHTTPPage(url,data) dim Http set Http=server.createobject("MSXML2.SERVERXMLHTTP.3.0") Http.open "POST",url,false Http.setRequestHeader "Content-Type", "application/x-www-form-urlencoded" Http.send(data) if Http.readystate<>4 then exit function End if PostHTTPPage=bytesToBSTR(Http.responseBody,"UTF-8") set http=nothing if err.number<>0 then err.Clear End function '提交數(shù)據(jù) dim postdata, strTest postdata="appid=&appid&"&module="&module&"&order="&order&"&sign="&sign strTest=PostHTTPPage(apiurl,postdata) '返回結(jié)果 response.write(strTest) response.end
7.2、接口二:快遞公司+快遞單號(hào)
'設(shè)置參數(shù) dim apiurl, appid, appkey, module, company, order, sign apiurl="http://cha.ebaitian.cn/api/json" appid="1000xxxx' appkey="56cf61af4b7897e704f67deb88ae8f24" module="getExpressInfoNoSmart" company="shunfeng" order="247198050163" '簽名,SHA256 不可直接調(diào)用;函數(shù)參考地址:https://blog.csdn.net/yesoce/article/details/128546 sgin=SHA256("appid=&appid&"&module="&module&"&company="&company&"&order="&order&"&appkey="&appkey) '異步提交數(shù)據(jù) function PostHTTPPage(url,data) dim Http set Http=server.createobject("MSXML2.SERVERXMLHTTP.3.0") Http.open "POST",url,false Http.setRequestHeader "Content-Type", "application/x-www-form-urlencoded" Http.send(data) if Http.readystate<>4 then exit function End if PostHTTPPage=bytesToBSTR(Http.responseBody,"UTF-8") set http=nothing if err.number<>0 then err.Clear End function '提交數(shù)據(jù) dim postdata, strTest postdata="appid=&appid&"&module="&module&"&company="&company&"&order="&order&"&sign="&sign strTest=PostHTTPPage(apiurl,postdata) '返回結(jié)果 response.write(strTest) response.end
本文為「本站原創(chuàng)」,未經(jīng)我們?cè)S可,嚴(yán)謹(jǐn)任何人或單位以任何形式轉(zhuǎn)載或刊載本文章,我們保留依法追究侵權(quán)的權(quán)力!
微信聯(lián)系我們
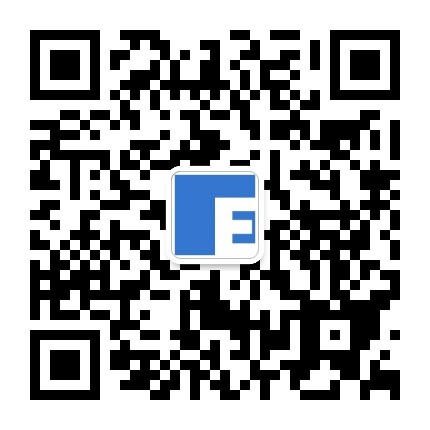
使用微信掃一掃
昵稱:億百天技術(shù)
公司:湖北億百天信息技術(shù)有限公司
電話:027-88773336
手機(jī):15342213852
郵箱:serviceebaitian.cn
我來(lái)說(shuō)兩句